When developing with Laravel, leveraging the power of Eloquent ORM can greatly enhance your application’s database interactions. Eloquent joins allow developers to build complex queries using elegant and expressive syntax. Mastering this aspect of Laravel improves the readability of the code and optimizes performance. Below, we delve into the intricacies of Eloquent joins, providing insights that will elevate your Laravel development skills. Keep reading to become proficient in creating advanced query statements.
Exploring the Basics of Eloquent Joins in Laravel
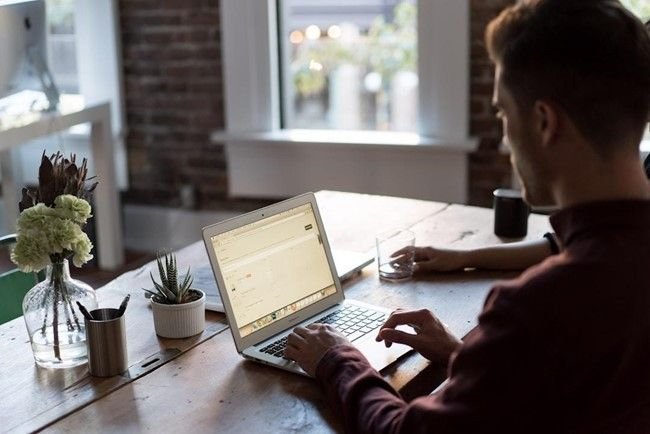
Eloquent ORM is integral to the Laravel framework, simplifying database operations with its Object-Relational Mapping capabilities. At the foundation, Eloquent joins connect and retrieve data from multiple database tables efficiently. This process requires understanding the relationships between tables and selecting the type of join that best fits the use case.
The common types of Eloquent join include inner, left, right, and cross joins. Each performs a specific operation on the tables involved, and choosing the appropriate join type is crucial for the intended results. This powerful feature allows developers to craft queries that would otherwise require complex SQL statements, drastically reducing development time.
Laravel’s Eloquent ORM offers a fluent API to construct joins, encapsulating the intricacies of raw SQL. Developers can chain methods to achieve the desired outcomes, providing a readable and maintainable codebase. For newcomers, grasping the basics of these joins is indispensable, as it sets the stage for more advanced database operations.
A simple Eloquent join query involves defining the base model, the related table, and the conditions for the join. This results in a seamless integration of data from multiple sources. Understanding the fundamental syntax and its implications is a stepping stone to mastering more complex Eloquent relationships.
Diving Deep into Inner Joins and Where Clauses
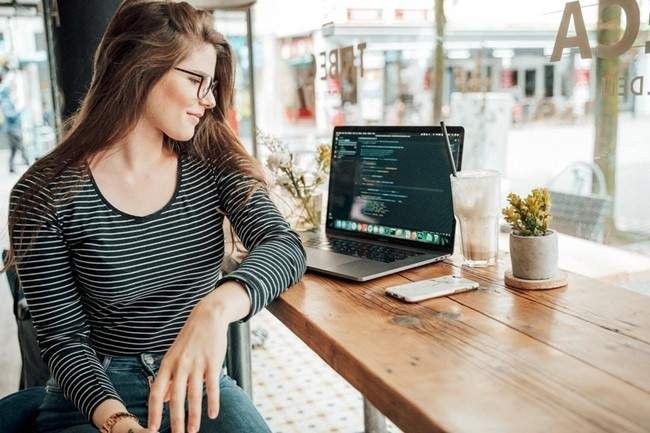
Inner joins are among the most commonly used types of joins in Eloquent. They retrieve records with matching values in both tables, effectively intersecting datasets based on specified conditions. An inner join brings a level of precision to database queries by ensuring that only relevant data is included in the results.
Alongside inner joins, ‘where’ clauses greatly enhance Eloquent’s querying capabilities. These clauses can be used to filter the join results based on specific conditions, further refining the dataset. When strategically combined, inner joins and where clauses create robust queries capable of handling complex data requirements.
Implementing a where clause in an Eloquent join is straightforward. The Laravel query builder provides intuitive methods such as ‘where’ and ‘where’ that can be effortlessly chained to the joint statement, allowing for agile development and easy code readability.
To further demonstrate the power of Eloquent joins, consider the example of filtering user data based on specific criteria. Using an inner join with a where clause, one can extract precise user information pertaining to multiple related tables, thus building feature-rich, data-driven applications with Laravel.
Leveraging Left, Right, and Cross Joins in Eloquent
Aside from inner joins, Eloquent supports left, right, and cross joins. A left join will include all records from the ‘left’ table and matched records from the ‘right’ table, filling in NULLs for unmatched pairs. This type of join is particularly helpful when extracting all records from one table with the corresponding matches from another.
Conversely, a right join mirrors the behavior of a left join but focuses on the ‘right’ table, ensuring that all its records are returned with the matches from the left. These joins are less commonly used but are indispensable in scenarios where ensuring the inclusion of all entries from a particular table is necessary.
Cross joins, on the other hand, combine each row from two tables, providing a Cartesian product of the sets. While this type of join is less common in everyday use due to the voluminous results it can produce, it serves specific purposes that can be beneficial in particular analytical contexts.
Utilizing each of these join types efficiently necessitates a solid understanding of their mechanics and implications on the dataset. By leveraging these joins, developers have the flexibility to construct versatile queries that align precisely with their data retrieval needs in Laravel’s Eloquent ORM.
Overall, mastering Eloquent joins in Laravel empowers developers to build efficient and readable queries that seamlessly integrate data from multiple tables. By understanding and applying various join types, developers can create flexible, performance-optimized database operations tailored to their application’s needs.